Hey there! Have you heard about serverless function with NodeJS on AWS Lambda? If you’re a developer, you probably have. But if not, don’t worry – I’m here to help.
In this blog post, we’re going to explore what serverless computing is, what AWS Lambda is, and how it works. Then, we’ll dive into a fun and practical tutorial on how to create a password generator using NodeJS on AWS Lambda. This real-world example will help you get started with serverless computing and AWS Lambda, and show you just how easy it can be to create your own serverless function. So, let’s get started!
What is Serverless computing ?
Serverless is a buzzword in technology, but it’s not just hype. Serverless programming offers many benefits over traditional server-based development, enabling developers to focus on the code they write rather than having to worry about infrastructure and scalability.
What is AWS Lambda ?
AWS Lambda is a serverless computing service provided by Amazon Web Services (AWS). It allows users to run code without provisioning or managing servers, which eliminates the need for traditional infrastructure management tasks such as server setup, scaling, and maintenance. Instead, Lambda automatically scales and provisions the required compute resources to run the code in response to incoming requests or events.
Lambda supports several programming languages, including Python, Node.js, Java, C#, Go, and Ruby. Users can write and upload their code to Lambda, define triggers that activate the function, and configure runtime settings such as memory and timeout limits. Lambda functions can be triggered by a wide range of event sources, such as API Gateway requests, S3 bucket updates, and CloudWatch Events.
AWS Lambda charges users based on the number of requests, the duration of the code execution, and the amount of memory used by the function. This usage-based pricing model allows users to pay only for the compute resources they consume, without the need to reserve or prepay for capacity.
Lambda is commonly used to build serverless applications, backend APIs, data processing pipelines, and event-driven architectures. It integrates with several other AWS services, such as DynamoDB, S3, and Kinesis, to create fully serverless solutions.
How AWS lambda works ?
AWS Lambda architecture is designed to provide a highly scalable, event-driven computing service that can execute code in response to various triggers or events. The main components of the AWS Lambda architecture include:
-
Function code: The function code is the actual code that the AWS Lambda service runs in response to triggers or events. It can be written in several programming languages, including Node.js, Python, Java, C#, Go, and Ruby.
-
Function handler: The function handler is a method within the function code that AWS Lambda invokes to start the execution of the code. It is responsible for processing the incoming events or triggers and generating the corresponding output.
-
Event sources: Event sources are the triggers that cause the AWS Lambda service to execute the function code. AWS Lambda supports a wide range of event sources, such as API Gateway, S3 bucket changes, and CloudWatch events.
-
Execution environment: AWS Lambda provides a managed execution environment that runs the function code when triggered by an event source. The execution environment automatically manages the compute resources required to execute the code, such as CPU, memory, and network bandwidth.
-
Security: AWS Lambda provides several security features, such as IAM roles and policies, to control access to AWS resources and to secure the function code.
-
Monitoring and logging: AWS Lambda generates logs and metrics that help users monitor the function execution and troubleshoot issues. The service integrates with several other AWS services, such as CloudWatch Logs and X-Ray, to provide a comprehensive monitoring and logging solution.
-
Deployment package: The deployment package is a zip archive that contains the function code, its dependencies, and any other required artifacts. Users can create the deployment package manually or use a deployment pipeline to automate the process.
Let’s get started!
Now that we have a better understanding of serverless computing and AWS Lambda, let’s get started with creating our own serverless function! In this tutorial, we’ll be walking through the steps to create a password generator using NodeJS on AWS Lambda. This password generator function will be triggered by a test event, and it will generate a random password for the user. By following along with this tutorial, you’ll learn how to create a serverless function, set up an API Gateway to trigger it, and test it out in a real-world scenario. So, grab a cup of coffee and let’s dive in!
Setup AWS Account
The first step in creating a serverless function on AWS Lambda is to create an AWS account. If you already have an account, you can skip this step. Otherwise, you can sign up for a free account on the AWS website.
Create a New Lambda Function
- Go to the AWS Lambda service in the AWS Management Console.
- Click the “Create function” button.
- Choose “Author from scratch”.
- Give your function a name, such as “password-generator”.
- Choose “Node.js 18.x” as the runtime.
- Live the default change execution role set to Create a new role with basic lambda permissions
- Click the “Create function” button.
Your new Lambda function is now created and ready to be edited.
Write the Function Code
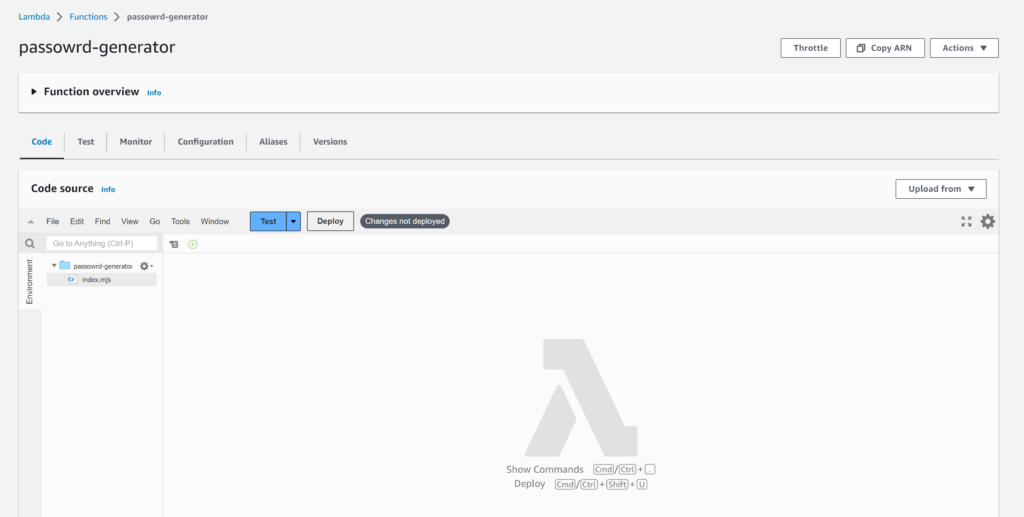
- Click the “Code” tab.
- In the “index.mjs” file, replace the default code with the following code
export const handler = async (event) => {
const length = parseInt(event.queryStringParameters.length) || 12;
const chars = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789";
let password = "";
for (let i = 0; i < length; i++) {
password += chars.charAt(Math.floor(Math.random() * chars.length));
}
return {
statusCode: 200,
headers: {
"Content-Type": "application/json",
"Access-Control-Allow-Origin": "*"
},
body: JSON.stringify({ password: password })
};
};
This code defines a Lambda function that generates a random password of a specified length. The password length is specified in the “length” query parameter of the HTTP request.
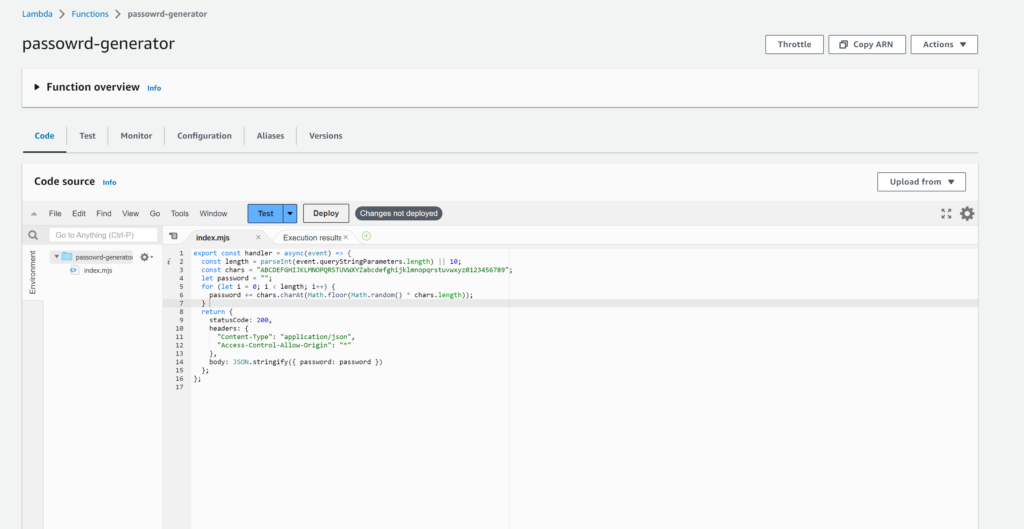
Deploy the Function
- Click the “Deploy” button at the top of the screen.
Your Lambda function is now deployed and ready to be tested.
Test the Function
- Go to the “Test” tab in the AWS Lambda console.
- Create a new test event by giving a name with the following JSON payload
{
"queryStringParameters": {
"length": "30"
}
}
This payload specifies that we want a password of length 30.
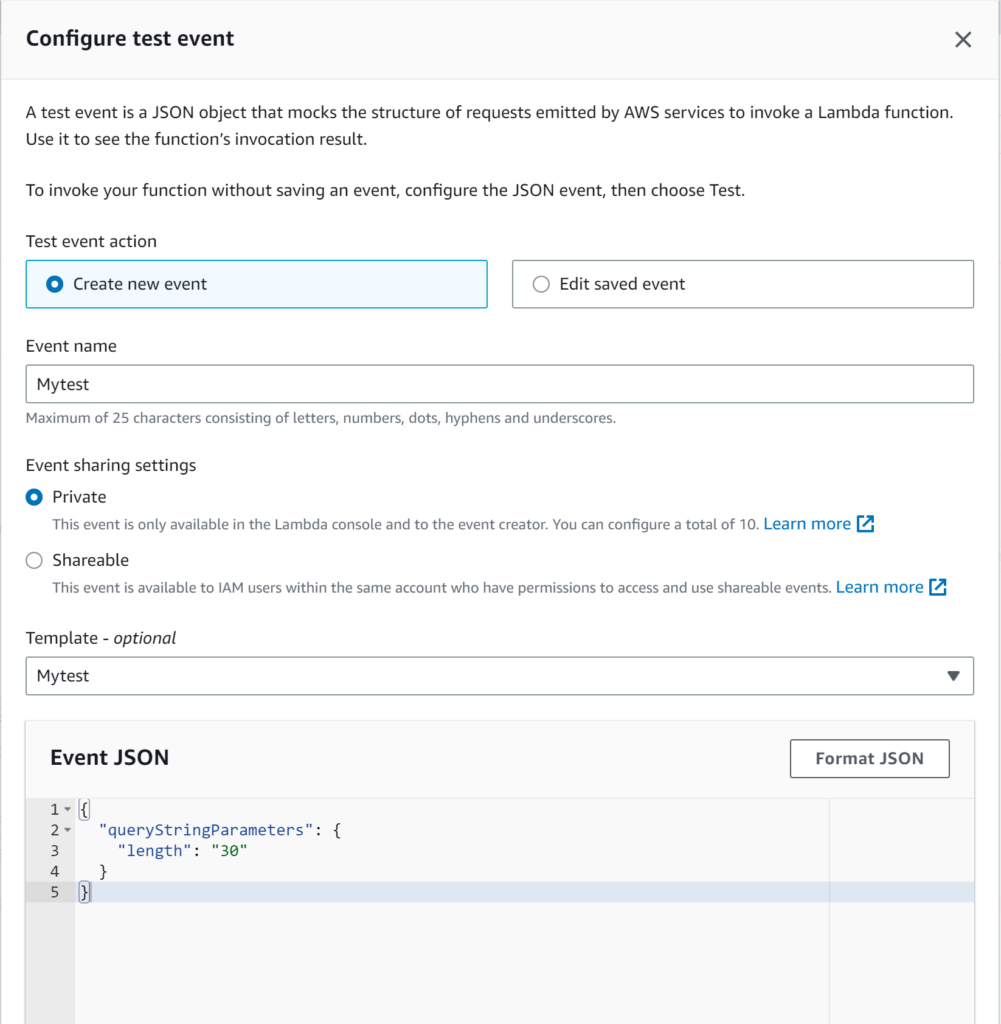
- Scroll to the bottom and click the save button
- Click the “Test” button.
The Lambda function should now generate a random password of length 30 and return it in a JSON format.
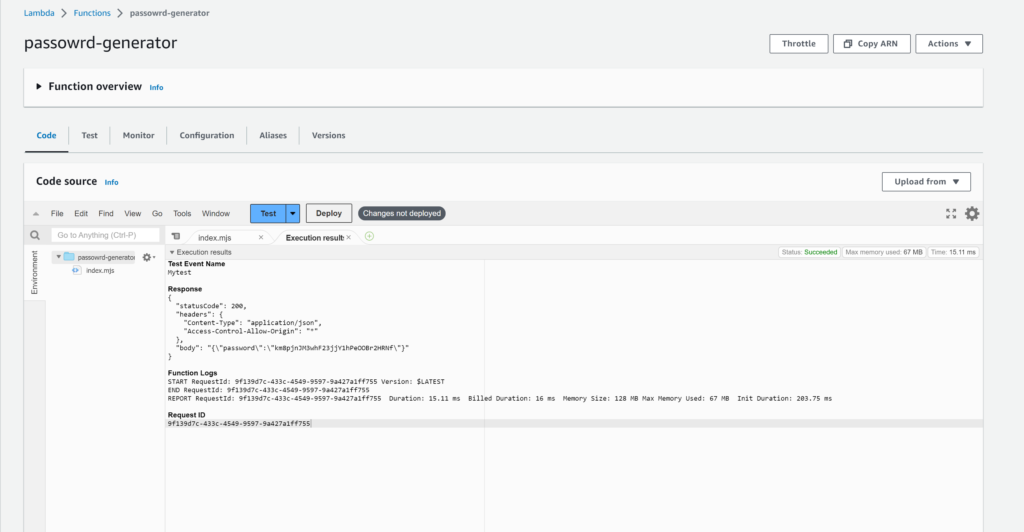
Congratulations! You just tested successfully your lambda function.
Conclusion
In conclusion, we have learned about serverless computing, its benefits, and how AWS Lambda is a popular serverless computing service offered by Amazon Web Services. We have also discussed the basic architecture of AWS Lambda and how it works. We then went on to create a serverless function with Nodejs on AWS Lambda by following a step-by-step guide.
With the popularity of serverless computing, AWS Lambda offers an easy and cost-effective way to run your code without managing servers. By leveraging the managed execution environment, you can focus on writing your code and let AWS handle the underlying infrastructure and management tasks.
As a real-world example, we have created a password generator function using Nodejs on AWS Lambda.